7. Buttons in Jetpack Compose
- Ranjith kumar
- Jul 26, 2021
- 2 min read
Updated: Sep 30, 2021
In Jetpack Compose buttons, you need to give two arguments for buttons. The first argument as onClick callback and another one is your button text element. You can add a Text-Composable or any other Composable as child elements of the Button.
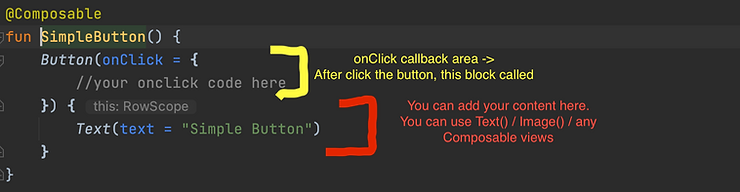
1. Simple Button:
@Composable
fun SimpleButton() {
Button(onClick = {
//your onclick code here
}) {
Text(text = "Simple Button")
}
}
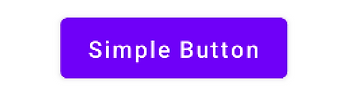
2. Button with custom color:
@Composable
fun ButtonWithColor(){
Button(onClick = {
//your onclick code
},
colors = ButtonDefaults.buttonColors(backgroundColor = Color.DarkGray))
{
Text(text = "Button with gray background",color = Color.White)
}
}

3. Button with multiple text:
@Composable
fun ButtonWithTwoTextView() {
Button(onClick = {
//your onclick code here
}) {
Text(text = "Click ", color = Color.Magenta)
Text(text = "Here", color = Color.Green)
}
}
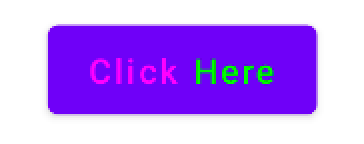
4. Button with Icon:
@Composable
fun ButtonWithIcon() {
Button(onClick = {}) {
Image(
painterResource(id = R.drawable.ic_cart),
contentDescription ="Cart button icon",
modifier = Modifier.size(20.dp))
Text(text = "Add to cart",Modifier.padding(start = 10.dp))
}
}
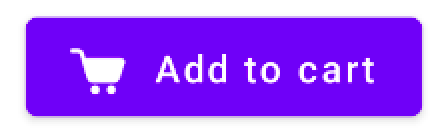
5. Button with shapes
Rectangle Shape:
@Composable
fun ButtonWithRectangleShape() {
Button(onClick = {}, shape = RectangleShape) {
Text(text = "Rectangle shape")
}
}
Round Corner Shape:
@Composable
fun ButtonWithRoundCornerShape() {
Button(onClick = {}, shape = RoundedCornerShape(20.dp)) {
Text(text = "Round corner shape")
}
}
Cut Corner Shape:
@Composable
fun ButtonWithCutCornerShape() {
//CutCornerShape(percent: Int)- it will consider as percentage
//CutCornerShape(size: Dp)- you can pass Dp also.
//Here we use Int, so it will take percentage.
Button(onClick = {}, shape = CutCornerShape(10)) {
Text(text = "Cut corner shape")
}
}
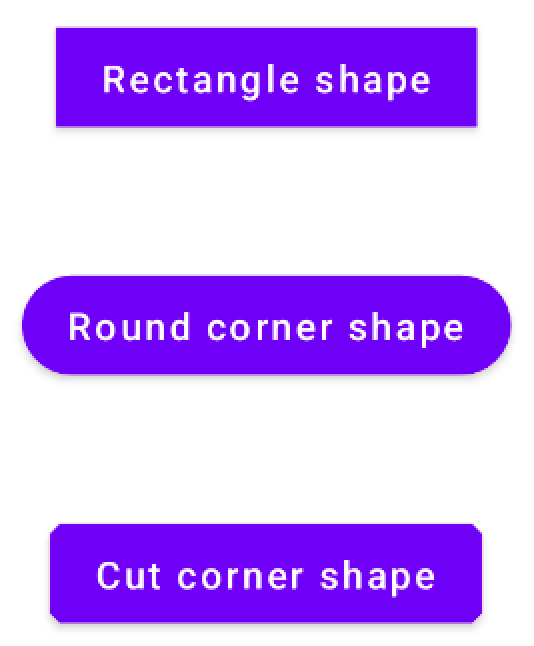
6. Button with Border:
@Composable
fun ButtonWithBorder() {
Button(
onClick = {
//your onclick code
},
border = BorderStroke(1.dp, Color.Red),
colors = ButtonDefaults.outlinedButtonColors(contentColor = Color.Red)
) {
Text(text = "Button with border", color = Color.DarkGray)
}
}

7. Button elevation:
@Composable
fun ButtonWithElevation() {
Button(onClick = {
//your onclick code here
},elevation = ButtonDefaults.elevation(
defaultElevation = 10.dp,
pressedElevation = 15.dp,
disabledElevation = 0.dp
)) {
Text(text = "Button with elevation")
}
}

Comentarios