14. Jetpack Compose Progress Indicator (ProgressBar)
- Ranjith kumar
- Jul 19, 2021
- 2 min read
Updated: Oct 4, 2021
Progress Indicator is a widget to indicate some actions are in progress to the user.
For long-time operations such as file downloading, uploading, API calls, we can inform the user to wait with the help of this component.
If you are an Android developer - It's a ProgressBar
Types of Progress Indicators available in Jetpack Compose
LinearProgressIndicator
CircularProgressIndicator
LinearProgressIndicator
It's used to display a progress in a linear line.
It supports two modes to represent progress: determinate, and indeterminate.
1. Indeterminate progress:
If you don't know how long your action will take then use indeterminate mode. It will run forever.
Example:
LinearProgressIndicator()
Output:
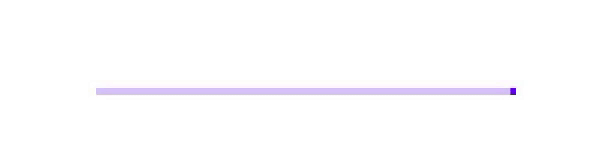
2. Determinate progress:
Use determinate mode for the progress bar when you want to show that a specific quantity of progress has occurred. For example, the percent remaining of a file being retrieved.
You need to give the progress value as a parameter.
It should be 0.0 to 1.0.
Example:
LinearProgressIndicator(progress = 0.7f) //70% progress
Output:

3. Custom LinearProgressIndicator:
You can customize the size, color of the progress, background color etc.
Example:
@Composable
private fun CustomLinearProgressBar(){
Column(modifier = Modifier.fillMaxWidth()) {
LinearProgressIndicator(
modifier = Modifier
.fillMaxWidth()
.height(15.dp),
backgroundColor = Color.LightGray,
color = Color.Red //progress color
)
}
}
Output:

CircularProgressIndicator
It used to display the progress in a circular shape. It also supports two modes: determinate, and indeterminate.
1. Indeterminate progress:
Example:
CircularProgressIndicator()
Output:
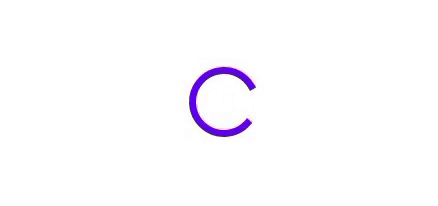
2. Determinate progress:
Example:
CircularProgressIndicator(progress = 0.75f) //75% progress
Output:
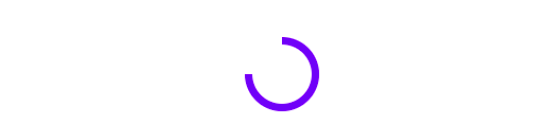
It will not animate by default. But we can animate with the help of Jetpack Compose animations.
Refer our blog about Jetpack Compose animations:
3. Determinate progress with Animation:
Example:
//Determinate (based on input)
@Composable
private fun CircularProgressAnimated(){
val progressValue = 0.75f
val infiniteTransition = rememberInfiniteTransition()
val progressAnimationValue by infiniteTransition.animateFloat(
initialValue = 0.0f,
targetValue = progressValue,animationSpec = infiniteRepeatable(animation = tween(900)))
CircularProgressIndicator(progress = progressAnimationValue)
}
Output:
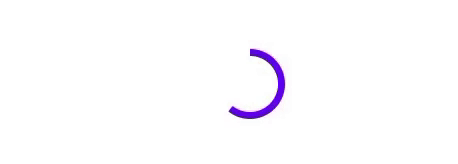
4.Custom CircularProgressIndicator
We can customize the progress indicator color, stroke width, width and height.
Example:
//Indeterminate (Infinity)
@Composable
private fun CustomCircularProgressBar(){
CircularProgressIndicator(
modifier = Modifier.size(100.dp),
color = Color.Green,
strokeWidth = 10.dp)
}
Output:
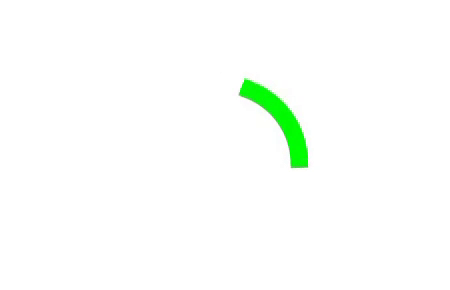
Source code:
Official documentation:
Comentarios