11. TopAppBar and Bottom Navigation with Scaffold
- Ranjith kumar
- Jul 22, 2021
- 3 min read
Updated: Dec 12, 2021
What's Scaffold? It allows you to implement a UI with the basic Material Design layout structure. You can add the following widgets with the help of Scaffold,
TopAppBar (Toolbar)
Floating Action Button (FAB)
Drawer Menu
Bottom Navigation
Structure of Scaffold:
Scaffold(
topBar = { //your top bar },
floatingActionButton = {//your floating action button},
drawerContent = { //drawer content },
content = { //your page content},
bottomBar = { //your bottom bar composable }
)
Simple example:
@Composable
fun ScaffoldSample() {
val scaffoldState = rememberScaffoldState(rememberDrawerState(DrawerValue.Closed))
Scaffold(
scaffoldState = scaffoldState,
topBar = { TopAppBar(title = {Text("Top App Bar")},backgroundColor = MaterialTheme.colors.primary) },
floatingActionButtonPosition = FabPosition.End,
floatingActionButton = { FloatingActionButton(onClick = {}){
Icon(imageVector = Icons.Default.Add, contentDescription = "fab icon")
} },
drawerContent = { Text(text = "Drawer Menu 1") },
content = { Text("Content") },
bottomBar = { BottomAppBar(backgroundColor = MaterialTheme.colors.primary) { Text("Bottom App Bar") } }
)
}
We add the topBar, floatingActionButton, drawer, bottomBar.
Output:
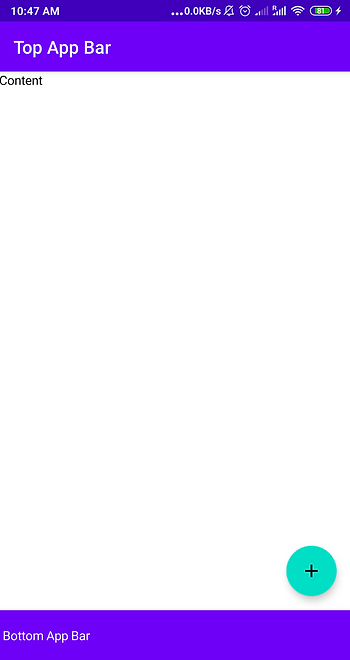
TopAppBar
Attributes of TopAppBar
title - This is use to represent the title for our action bar.
navigationIcon - This icon to be displayed at the start of the app bar.
backgroundColor - This is use to represent backgroundColor of our top app bar.
contentColor - This color is use to give color to our contents of our action bar.
elevation - This is use to give elevation to our top app bar.
If you are an Android developer, it's Toolbar
Structure of TopAppBar
TopAppBar(
title = {
//composable function for title
},
navigationIcon = {
//composable function for leading icon
},
backgroundColor = //color code,
contentColor = //color code,
elevation = //your elevation value in Dp
)
Sample code:
@Composable
fun ScaffoldWithTopBar() {
Scaffold(
topBar = {
TopAppBar(
title = {
Text(text = "Top App Bar")
},
navigationIcon = {
IconButton(onClick = {}) {
Icon(Icons.Filled.ArrowBack, "backIcon")
}
},
backgroundColor = MaterialTheme.colors.primary,
contentColor = Color.White,
elevation = 10.dp
)
}, content = {
Column(
modifier = Modifier
.fillMaxSize()
.background(Color(0xff8d6e63)),
verticalArrangement = Arrangement.Center,
horizontalAlignment = Alignment.CenterHorizontally
) {
Text(
text = "Content of the page",
fontSize = 30.sp,
color = Color.White
)
}
})
}
In this example we set the title and navigationIcon (back button) and also we set the color and elevation. In content area, we set the Column with one Text().
Output:
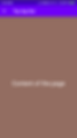
Title customization:
You can customize the title area like this,
title = {
Row() {
Text(
text = "Title 1",
fontSize = 30.sp,
color = Color.Red
)
Text(
text = "Title 2",
fontSize = 30.sp,
color = Color.White
)
}
}
title - It accept any composable. Here we use the Row() with two different Text()
Output:

BottomNavigation
We can show bottom menu items with help of BottomNavigation. It make it easy for users to explore and switch between top-level views in a single tap.
If you are an Android developer, It's BottomNavigationView
Structure of BottomNavigation:
BottomNavigation(
modifier = //your modifier ,
backgroundColor = //color code,
contentColor = //color code,
elevation = //your elevation value in Dp
) {
BottomNavigationItem1()
BottomNavigationItem2()
BottomNavigationItem3()
.......
}
Structure of BottomNavigationItem:
BottomNavigationItem(icon = {
//composable function for menu icon
},
label = { //composable function for menu title},
selected = //mutableState boolean for highlight,
onClick = {
//menu item click event
})
BottomNavigation sample code
Step 1 : Create a composable function for BottomNavigation
@Composable
fun BottomBar() {
val selectedIndex = remember { mutableStateOf(0) }
BottomNavigation(elevation = 10.dp) {
BottomNavigationItem(icon = {
Icon(imageVector = Icons.Default.Home,"")
},
label = { Text(text = "Home") },
selected = (selectedIndex.value == 0),
onClick = {
selectedIndex.value = 0
})
BottomNavigationItem(icon = {
Icon(imageVector = Icons.Default.Favorite,"")
},
label = { Text(text = "Favorite") },
selected = (selectedIndex.value == 1),
onClick = {
selectedIndex.value = 1
})
BottomNavigationItem(icon = {
Icon(imageVector = Icons.Default.Person,"")
},
label = { Text(text = "Profile") },
selected = (selectedIndex.value == 2),
onClick = {
selectedIndex.value = 2
})
}
}
Step 2: Add into Scaffold
@Composable
fun ScaffoldWithBottomMenu() {
Scaffold(bottomBar = {BottomBar()}
) {
//content area
Box(modifier = Modifier
.background(Color(0xff546e7a))
.fillMaxSize())
}
}
Output:
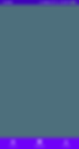
Source code:
https://github.com/JetpackCompose/Jetpack-Compose-Samples/blob/master/JetPackComposeSamples/app/src/main/java/net/jetpackcompose/composetext/activities/ActivityScaffold.kt