5. TextStyle in JetPack Compose
- Ranjith kumar
- Jul 27, 2021
- 2 min read
Updated: Dec 12, 2021
Introduction:
Text plays important role in mobile/web applications. We can present the details to the user using Text. Let's assume If we use the same font, same color, same size for this entire blog it doesn't look good and users can't able to understand. So we need to style this widget using the TextStyle option to enhance the user experience.
In Jetpack Compose, we can use the following options for applying a TextStyle()
Text(
text = "Hello World",
style = TextStyle(
color = Color.Red,
fontSize = 16.sp,
fontFamily = FontFamily.Monospace,
fontWeight = FontWeight.W800,
fontStyle = FontStyle.Italic,
letterSpacing = 0.5.em,
background = Color.LightGray,
textDecoration = TextDecoration.Underline
)
)
1.Text Color:
Text(
text = "Text with Color",
style = TextStyle(color = Color.Red)
)
2. Background Color:
Text(
text = "Text with Background Color",
style = TextStyle(background = Color.Yellow)
)
3. Shadow:
Text(
text = "Text with Shadow",
style = TextStyle(
shadow = Shadow(
color = Color.Black,
offset = Offset(5f, 5f),
blurRadius = 5f
)
)
)
4. Font Family:
You can use following system fonts or your custom font.
val Default: SystemFontFamily = DefaultFontFamily()
val SansSerif = GenericFontFamily("sans-serif")
val Serif = GenericFontFamily("serif")
val Monospace = GenericFontFamily("monospace")
val Cursive = GenericFontFamily("cursive")
Example:
Text(
text = "Text with custom font",
style = TextStyle(fontSize = 20.sp, fontFamily = FontFamily.Cursive)
)
5. Font Size:
Text(
text = "Text with big font size",
style = TextStyle(fontSize = 30.sp)
)
6. Font Style:
You can use either FontStyle.Normal or FontStyle.Italic
Text(
text = "Text with Italic text",
style = TextStyle(fontSize = 20.sp, fontStyle = FontStyle.Italic)
)
Output:
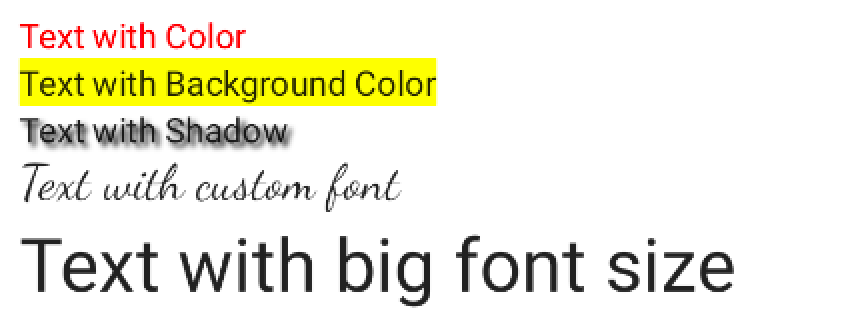
7. Text Decoration:
You can use either TextDecoration.Underline or TextDecoration.LineThrough
TextDecoration.Underline - Draws a horizontal line below the text. TextDecoration.LineThrough - Draws a horizontal line over the text.
@Composable
fun TextDecorationStyle() {
Column {
Text(
text = "Text with Underline",
style = TextStyle(
color = Color.Black, fontSize = 24.sp,
textDecoration = TextDecoration.Underline
)
)
Text(
text = "Text with Strike",
style = TextStyle(
color = Color.Blue, fontSize = 24.sp,
textDecoration = TextDecoration.LineThrough
)
)
}
}
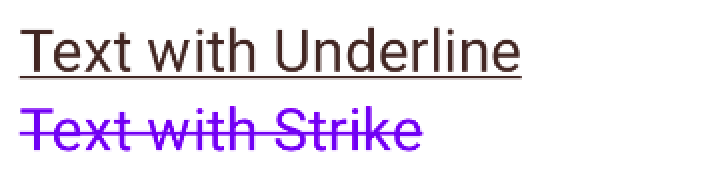
Typography:
From MaterialTheme, we can reuse the default typography. They customize the textstyle() with various text sizes.
Following options available in material theme typography:
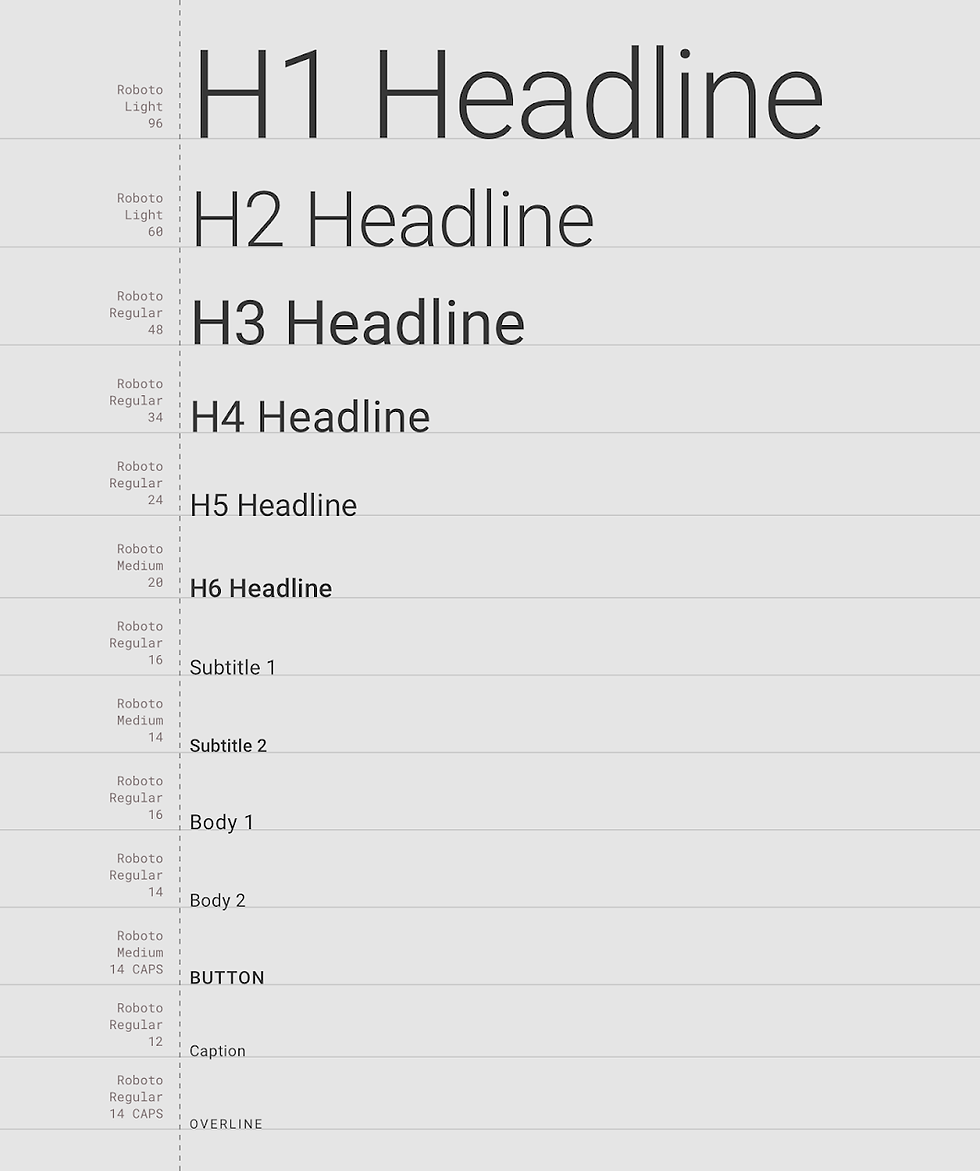
Sample code:
@Composable
fun TextHeadingStyle() {
Column(
modifier = Modifier
.fillMaxWidth()
.background(Color.Green)
) {
Text(
text = "Heading 3",
style = MaterialTheme.typography.h3
)
Text(
text = "Heading 4",
style = MaterialTheme.typography.h4
)
Text(
text = "Heading 5",
style = MaterialTheme.typography.h5
)
}
}
Output:
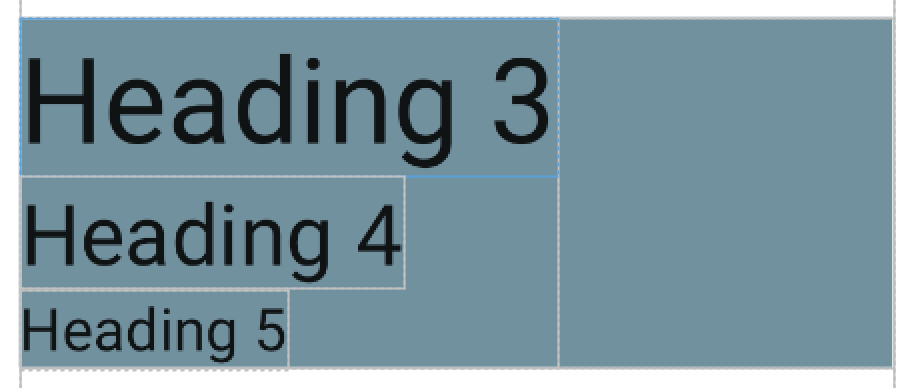
good series of tutorial ! just a suggestion that at the end of each tutorial , pls have a link for the next one in the sequence. I need to go back to the main page again and find the next one.